Understanding Threads in Computing: Importance and Types
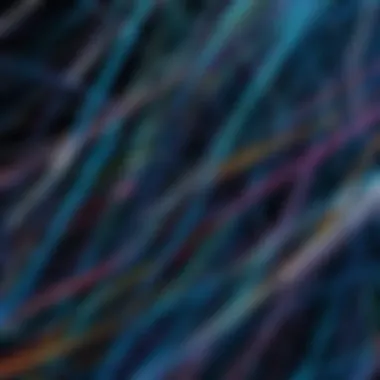
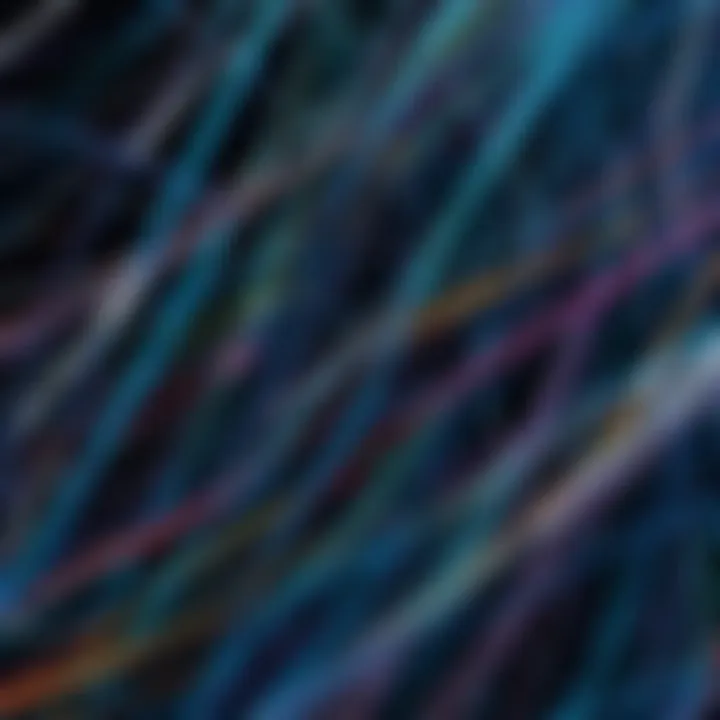
Intro
Threads in computing can be considered the backbone of modern software design. They allow applications to perform multiple tasks at the same time, enhancing overall performance and responsiveness. Understanding threads requires knowledge of several key concepts, including their definition, types, and significance in concurrent processing environments. This section provides a thorough introduction to threads and their roles in software and hardware, setting the stage for deeper exploration.
Performance Metrics
Performance is a crucial aspect when discussing threads in computing. It encompasses several key factors that directly impact how well an application utilizes threads and ultimately its efficiency. These metrics can be powerful indicators of a thread's effectiveness.
Benchmarking Results
Benchmarking results typically demonstrate the performance improvements that threading can provide. Various tools, such as Apache JMeter or LoadRunner, are available for testing applications that implement threading. When benchmark tests capture data from threaded vs. non-threaded executions, developers can get clear insights about how concurrency impacts different tasks.
Speed and Responsiveness
In terms of speed, threads can dramatically improve an application's response time. When a program uses multiple threads, it can handle user interfaces while simultaneously processing backend tasks. For instance, a video game can render graphics in real time while processing player inputs and game mechanics. This segregation allows users to have smoother experiences, reducing lag and improving interactions.
Usability and User Experience
Usability is another important consideration for developers who utilize threading in their applications. Although threads can enhance performance, they can also lead to complexity in installation and overall user experience design.
Ease of Installation and Setup
From a user standpoint, the installation of threaded applications should be as seamless as possible. If a threaded application is complicated to set up, potential users may become frustrated. Good practice involves providing clear documentation and utilizing installation wizards to guide users through the process. This ensures that users of varying technical skills can successfully install the software.
Interface Design and Navigation
Interface design plays a vital role in how users interact with threaded applications. A well-designed interface should clearly represent the state of ongoing threads and allow users to navigate easily without confusion. Proper design minimizes potential user errors and enhances satisfaction.
"Understanding how threads work is crucial not only for developers but also for users who demand efficient and responsive applications."
Intro to Threads
Threads are fundamental to understanding modern computing. They enable multiple tasks to be executed simultaneously, improving performance and responsiveness in software applications. In this article, we explore the concept of threads, their significance in various computing processes, and how they have transformed software architecture. By delving into thread management, synchronization challenges, and real-world applications, IT professionals and tech enthusiasts will gain valuable insight into this essential topic.
Defining a Thread
A thread is the smallest unit of processing that can be scheduled by an operating system. In simpler terms, it is a lightweight process that can run concurrently with other threads. Each thread has its own register set and stack space, yet shares memory and resources with other threads within the same process. This shared memory model facilitates communication and data sharing among threads but also introduces complexities related to synchronization and data integrity.
Threads can be categorized as either user-level or kernel-level, impacting how they are managed and scheduled. User threads are managed by a user-level library and are invisible to the operating system. Conversely, kernel threads are managed by the operating system itself, allowing better resource allocation and management by leveraging kernel-level scheduling capabilities.
Historical Context
The concept of threads emerged in the 1960s and 1970s alongside the development of multitasking operating systems. Earlier computing systems ran single tasks sequentially, limiting performance and efficiency. As demand for more responsive and efficient applications grew, developers began to explore concurrency. The evolution of threading coincided with advancements in computer architecture, particularly the shift from single-core to multi-core processors.
In the mid-1980s, the introduction of systems like UNIX and POSIX paved the way for implementing threads in operating systems. These frameworks established a foundation for thread management, allowing developers to create software that could leverage threading for performance gains. Today, threads are integral to almost every software application, from web servers to real-time systems, shaping the landscape of modern computing.
The Importance of Threads in Computing
Threads play a crucial role in the field of computing, providing a framework for multitasking within a single process. This section elaborates on why threads are so important in contemporary computing environments, especially as applications become more complex and performance demands increase.
Performance Optimization
In modern computing, performance optimization is of paramount importance. Threads allow applications to perform multiple tasks simultaneously, significantly enhancing responsiveness and efficiency. Utilizing threads enables a program to break down its workload into smaller units of execution, which can then be processed concurrently.
For instance, in a web browser, one thread could manage the user interface while another processes data from the internet. This division of labor not only leads to smoother user experiences but also maximizes resource utilization.
- Concurrency: Threads facilitate executing multiple operations at once, improving the overall throughput of applications.
- Responsiveness: By delegating tasks to separate threads, applications can remain responsive to user input while performing background operations.
Resource Management
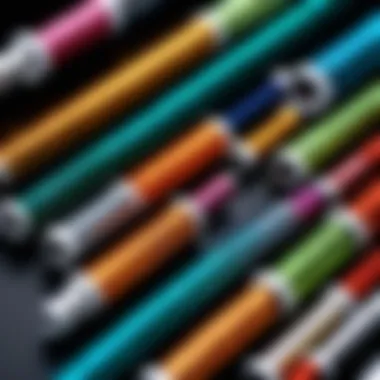
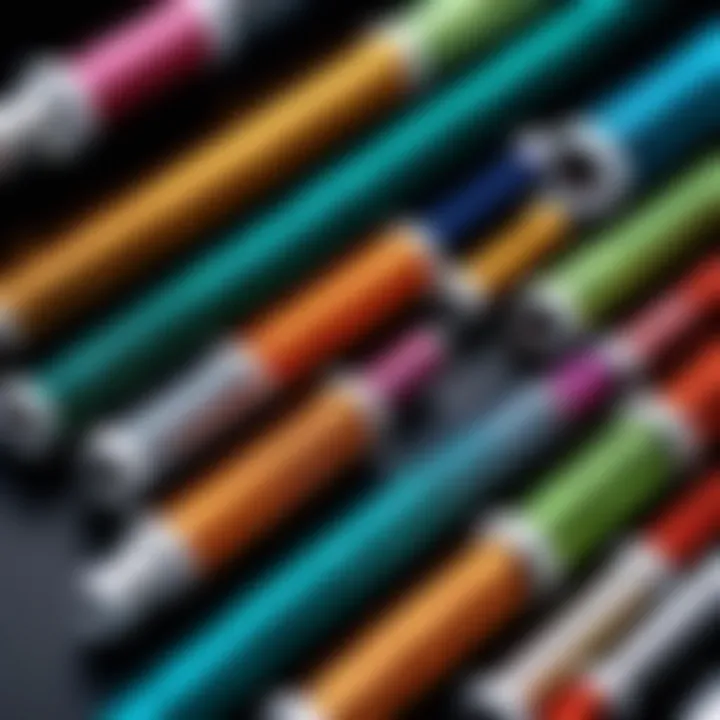
Threads also play a vital role in effective resource management. They allow for the optimal use of CPU and memory resources in a computing system. Through shared resources, such as memory space, threads can communicate more efficiently than separate processes. This means lighter overhead and faster context-switching.
Moreover, correct resource management is necessary to avoid overloading a system. Thread pools, for example, can manage a fixed number of active threads at any given time, thereby preventing resource exhaustion and improving stability.
- Lower Overheads: Threads share the same address space, allowing for quicker data exchange when compared to separate processes.
- Scalability: As applications grow more complex, using threads makes it easier to scale resources without significant re-engineering of the software's structure.
In summary, threads are indispensable in optimizing performance and managing resources efficiently in computing. They bridge the gap between complexity and effective performance, crucially impacting how software operates in an ever-evolving technological landscape.
"Efficient thread management is central to software performance in modern computing environments."
For further insights, you might want to explore Wikipedia) or Britannica for broader context on threading.
Types of Threads
Threads play a pivotal role in the efficient functioning of modern computing systems. Understanding the different types of threads is crucial for developers and IT professionals as it directly impacts performance, resource utilization, and programmability. This section delves into the three predominant types of threads: User threads, Kernel threads, and Hybrid threads. Each type has unique attributes, advantages, and considerations that influence how applications are designed and executed.
User Threads
User threads are created and managed at the user level, often by threading libraries such as POSIX Threads (pthreads). They are not recognized by the operating system's kernel. User threads allow for greater control over execution and scheduling and can be implemented without incurring the overhead of system calls to the kernel. This leads to faster context switching since the user-level library handles it.
User threads are beneficial in several ways:
- Flexibility in management: Developers can employ various scheduling algorithms according to application requirements, enhancing performance.
- Lightweight: The absence of kernel involvement during execution reduces the resource overhead.
- Portability: User-level threads can be used across different operating systems as they do not rely on kernel support.
However, there are limitations. If a user thread blocks, the entire process may become unresponsive, as the kernel is unaware of the individual threads. This detachment makes it harder to utilize multi-core processors effectively.
Kernel Threads
Kernel threads are managed directly by the operating system’s kernel. The kernel is aware of each thread, allowing it to perform context switching and scheduling more efficiently based on thread priority and other factors. Most modern operating systems, such as Windows and Linux, utilize kernel threads.
The benefits of kernel threads include:
- True concurrency: The ability for the kernel to manage multiple threads means that they can run in parallel on multiple cores.
- Immediate responsiveness: If one thread blocks, others can continue executing as the kernel handles them independently.
- Simplified synchronization: The kernel provides built-in mechanisms for synchronization, reducing the complexity for developers.
Conversely, kernel threads introduce some overhead due to the increased management by the operating system. Each thread requires kernel resources, which can create performance bottlenecks under high-load scenarios.
Hybrid Threads
Hybrid threading models combine aspects of both user and kernel threads to maximize their advantages while minimizing the downsides. In this structure, user threads are mapped to kernel threads. This allows for efficient resource management while leveraging the flexibility of user-level threading.
The hybrid approach offers:
- Enhanced performance: Developers can prioritize user threads while still allowing the kernel to optimize resource usage effectively.
- Better resource utilization: It enables the system to balance between user demands and system-level management, making it easier to adapt to changing workloads.
- Scalability: Hybrid threading can grow with applications, providing a powerful method for improving throughput and responsiveness without a complete redesign.
Nevertheless, the hybrid model can be complex in terms of implementation. Developers need to carefully design their threading strategy to reap the benefits without facing the intricacies of debugging mixed threading models.
The Mechanisms Behind Thread Management
Understanding the mechanisms behind thread management is central in mastering how threads function within computing environments. Thread management not only involves the creation and termination of threads but also requires effective scheduling to maximize performance and resource utilization. A solid grasp of these concepts can lead to more efficient programming practices and enhanced application performance. This section delves into the crucial elements of thread management, highlighting its benefits and necessary considerations for developers.
Thread Creation and Termination
Thread creation is the initial step in utilizing threads for parallelism. In most programming environments, threads are created using a specific function or method that allocates necessary system resources. The process may differ based on the threading model and language used. For example, in Java, developers use the class or implement the interface to create a new thread. Understanding this process is essential, as improperly managed thread creation can lead to resource leaks or decreased performance.
Once a thread has completed its task, it must be properly terminated. This involves signaling the operating system that the thread's work is finished, releasing any resources it was using. If threads are not terminated correctly, they may continue to occupy system resources, leading to what is known as a "zombie" thread. This can affect overall system performance, as it increases the load on the system for managing these inactive threads.
Here are some key considerations regarding thread creation and termination:
- Resource Allocation: Ensure adequate resources are allocated at thread creation to avoid performance degradation.
- Lifecycle Management: Implement proper lifecycle management to prevent orphaned or zombie threads.
- Error Handling: Make provisions for error handling during thread execution to manage unexpected terminations gracefully.
Scheduling Algorithms
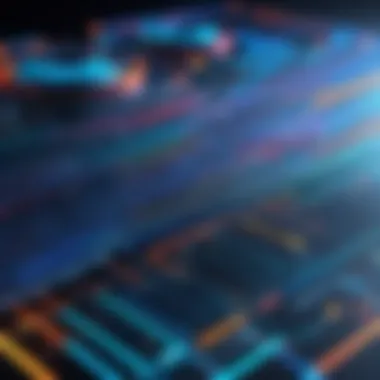
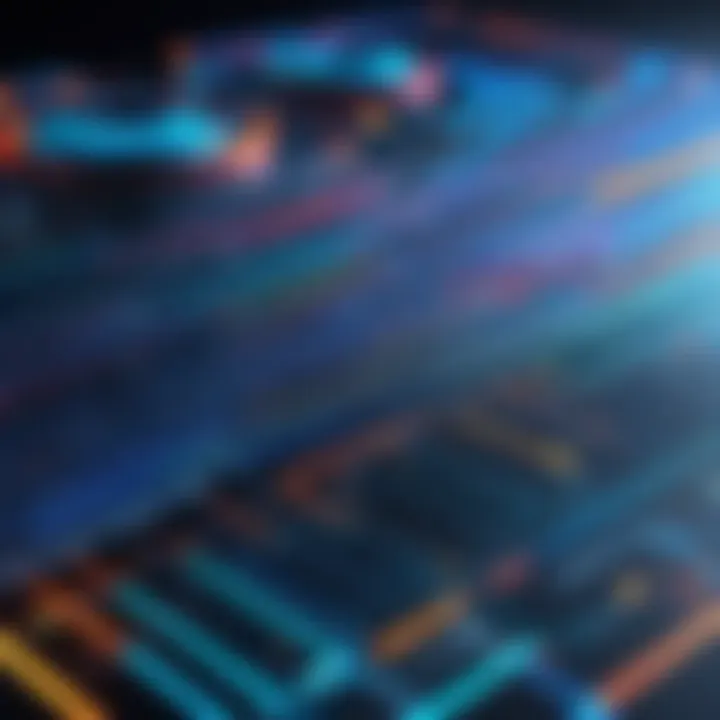
Scheduling algorithms play a vital role in managing how threads are prioritized for execution. The effectiveness of these algorithms significantly affects the performance of multi-threaded applications. They determine which thread runs at any given time, influencing responsiveness and efficiency. A common scheduling approach is preemptive scheduling, where the operating system can interrupt a currently running thread to allocate CPU time to another thread. This ensures more equal and efficient usage of processing power across multiple threads.
There are several types of scheduling algorithms, each with unique advantages:
- Round Robin: Allocates a fixed time slice for each thread, useful in time-sharing environments.
- Priority Scheduling: Threads are executed based on predefined priorities. High-priority threads receive the CPU first, which can be effective but risks starvation of lower-priority threads.
- Multilevel Queue Scheduling: Different queues are maintained for different priorities. Threads are placed in queues based on their priority and scheduling policies applied accordingly.
Understanding these algorithms is crucial as they can impact not only performance but also the overall responsiveness of applications. Choosing the right scheduling strategy depends on the specific needs of the application and the characteristics of the workload it processes.
"The efficiency of thread management hinges on effectively balancing the creation, termination, and scheduling of threads to optimize performance in computing environments."
Synchronization and Thread Safety
In the realm of multi-threaded programming, the concepts of synchronization and thread safety are of crucial importance. They help to manage the complexity that arises when multiple threads operate concurrently. Without proper synchronization, data integrity is at risk. Errors can happen, leading to unpredictable behaviors in applications. This section explores the significance of these topics, providing valuable insights for IT professionals and tech enthusiasts alike.
Understanding Race Conditions
Race conditions occur when two or more threads access shared data simultaneously and attempt to change it. If the threads do not synchronize their access, the end result can be inconsistent data. Imagine a scenario where two threads are updating a user's account balance. If one thread reads the balance while another is updating it, the final balance may not reflect both transactions correctly.
To avoid race conditions, developers implement synchronization mechanisms. Controlled access to shared resources ensures that only one thread can modify the data at a time, thus preserving data integrity. The overhead associated with managing these access controls must be balanced against the performance gains from multi-threading. In practice, it often requires careful design to minimize the impact on performance while ensuring safety.
Locking Mechanisms
Locking mechanisms are essential tools for achieving thread safety. These are constructs that ensure exclusive access to shared resources. There are several types of locks:
- Mutex (Mutual Exclusion): A mutex allows only one thread to access the critical section at any time. If a thread locks a mutex, other threads must wait until it releases the lock.
- Read/Write Locks: These differentiate between read and write access. Multiple threads can read concurrently, but writing requires exclusive access.
- Spinlocks: A lightweight locking mechanism where the thread repeatedly checks if a lock is available. This is useful when waiting times are expected to be minimal.
Locking can introduce performance overhead and lead to issues if not managed correctly, like contention and increased latency. Thus, understanding the right locking mechanism for the specific application is vital.
Deadlocks Explained
A deadlock is a situation where two or more threads are blocked forever, waiting for each other to release resources. In essence, each thread holds a resource needed by another thread, creating a cycle of dependency. The result is a complete halt in execution for the involved threads.
To prevent deadlocks, developers can employ several strategies:
- Resource Ordering: Define a strict order in which resources should be acquired, ensuring that cycles cannot form.
- Timeouts: Implement time limits on how long a thread will wait for a resource. If the limit is exceeded, the thread can back off and retry.
- Deadlock Detection: Regularly check for deadlocks during execution and take corrective actions, such as aborting one of the threads involved.
Using these strategies effectively can mitigate the risk of deadlocks, ensuring smoother execution in multi-threaded applications.
Proper synchronization and understanding locking mechanisms are foundational to prevent issues like race conditions and deadlocks. These practices not only enhance performance but also ensure robust application behavior.
Real-World Applications of Threads
Threads are critical in modern computing, forming the backbone of concurrent execution across a wide array of applications. The practical use of threads enables systems to utilize resources more effectively. As the demand for responsiveness and performance in software increases, understanding these real-world applications becomes essential for IT professionals and tech enthusiasts.
Concurrent Data Processing
Concurrent data processing exemplifies the power of multithreading. In environments where large volumes of data require processing simultaneously, threads allow for the division of tasks among different cores or processors. This leads to significant reductions in processing time and enhances overall system throughput. For instance, data parsing in applications such as Apache Kafka benefits from concurrent threads, enabling it to handle multiple data streams without latency.
Moreover, parallel processing frameworks such as Apache Spark leverage the thread model to efficiently distribute tasks across clusters. Each thread can handle a specific data subset, which allows for real-time analytics and big data processing. This efficiency is crucial in sectors ranging from finance to healthcare where timely data processing is critical.
Asynchronous Programming
Asynchronous programming relies heavily on threads to manage tasks without blocking the main execution thread. This approach is beneficial in user interfaces where long-running operations would hinder user experience if executed sequentially. Frameworks like Node.js use an event-driven model backed by asynchronous threads, allowing I/O-bound tasks to run concurrently while the application remains responsive.
An important aspect of asynchronous programming is the use of callbacks and promises. These elements enable developers to handle multiple operations simultaneously and improve application responsiveness. Furthermore, languages such as JavaScript incorporate asynchronous mechanisms to simplify writing concurrent code, allowing developers to focus on the business logic rather than managing concurrency complexities directly.
Multithreaded Web Servers
Multithreaded web servers are another prime illustration of threads in action. They handle multiple client requests concurrently, significantly improving throughput and response times. Servers such as Apache HTTP Server and Nginx use multithreading to cater to numerous simultaneous connections efficiently.
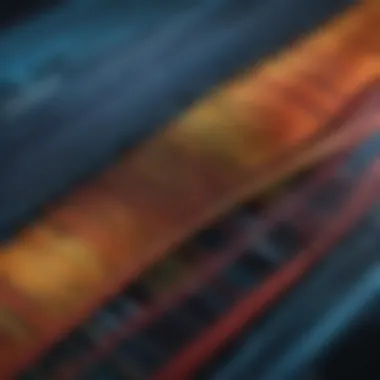
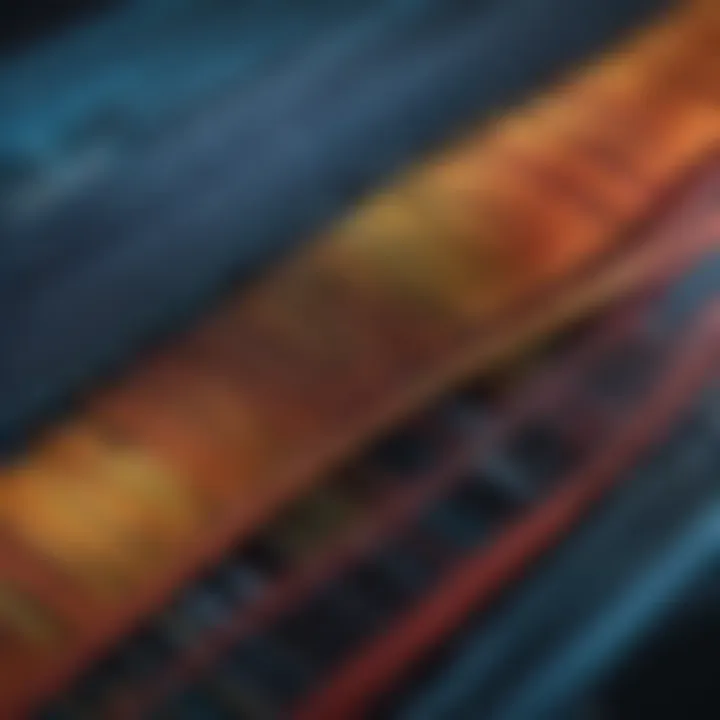
These servers employ a thread-per-request model, where a new thread is spawned for each incoming request. This model allows services to manage high traffic loads while maintaining performance. However, it comes with challenges such as the potential for resource contention and the need for careful thread management to avoid performance bottlenecks.
"Multithreaded web servers exemplify the balance of handling multiple tasks while ensuring optimal resource utilization, directly impacting user experience."
Challenges in Thread Programming
Thread programming introduces a complex landscape for developers, as it combines both opportunities and challenges. Understanding these challenges is vital for ensuring effective software performance and reliability.
One of the primary considerations is the difficulty of debugging multithreaded applications. Traditional debugging techniques used for single-threaded environments often fall short. This is largely due to the non-deterministic nature of thread execution, where timing and order of operations can affect the outcome. Consequently, bugs may not appear consistently, making them hard to isolate. Additionally, the synchronization issues that arise can introduce subtle errors.
Debugging Multithreaded Applications
Debugging in a multithreaded context highlights several key challenges. Here are some considerations:
- Race Conditions: These occur when two or more threads access shared data concurrently, resulting in unpredictable behavior. Identifying the source of a race condition can require sophisticated diagnostic tools and rigorous testing procedures.
- Thread Interference: This refers to situations where one thread’s operation negatively impacts another thread, leading to inconsistent data states. General debugging methods may not reveal interference until the application runs in a real-world scenario.
- Tool Limitations: While numerous debugging tools exist, many are primarily designed for single-threaded environments. Adapting them for multithreaded debugging is often not straightforward, and developers may find themselves relying on trial and error instead.
These factors emphasize the necessity of understanding the intricacies of thread behavior during the software development lifecycle. Proper training and experience are crucial for overcoming these obstacles and achieving successful multithreaded applications.
Performance Bottlenecks
Performance bottlenecks present another significant challenge in thread programming. As more threads are spawned, systems may face diminishing returns due to various factors:
- Context Switching: Each time a thread switches, the operating system must save the state of the current thread and load the state of the new one. This process consumes CPU cycles and can significantly degrade performance if not managed correctly.
- Resource Contention: When multiple threads compete for the same resources, it can lead to delays. This contention can become problematic with I/O operations as threads wait for access to disk, network, or memory resources.
- Excessive Thread Creation: Developers may overestimate the benefits of multithreading and spawn too many threads, leading to overhead that outweighs the performance gains. It’s crucial to find a balance between the number of threads and system capabilities.
Addressing performance bottlenecks often necessitates an understanding of both hardware and software constraints. Optimizing thread count, managing resources effectively, and using profiling tools for performance analysis are specific actions developers can take to mitigate these challenges.
"In multithreaded programming, effective design and testing strategies are as important as implementation."
Considering these challenges helps refine the approach to thread programming, ultimately leading to more resilient and efficient applications.
Future Trends in Threading Technology
The landscape of threading technology is continuously evolving. This section explores the emerging trends that shape the future of threading in computing. Understanding these trends is crucial for IT professionals and enthusiasts. As technology advances, threads are expected to play an even more pivotal role in performance optimization and resource management. Knowing what to anticipate helps in adapting to changes and harnessing new capabilities effectively.
Emerging Paradigms
In recent years, several paradigms have come to the forefront in threading technology. One prominent trend is data parallelism. This concept involves executing the same operation on different pieces of distributed data simultaneously. For instance, frameworks like TensorFlow utilize this approach extensively in machine learning operations, allowing for more efficient data processing.
Another paradigm gaining traction is actor model concurrency. This model simplifies thread management by using lightweight, isolated actors that communicate through message passing. It significantly reduces the complexity of multithreaded programming. Libraries and frameworks such as Akka implement this model, providing a powerful way to manage concurrent tasks.
Additionally, asynchronous programming has become increasingly relevant. Asynchronous models allow programs to perform tasks without blocking other operations. This is particularly useful in web development, where servers handle multiple client requests simultaneously. JavaScript’s and Python’s are prime examples of how these concepts are being applied.
Impact on Software Development
The emergence of these paradigms will inevitably influence software development practices. First, improved efficiency is a direct benefit. Developers can write more streamlined code that enhances system performance. As systems become more efficient, this can lead to cost savings in resource usage.
Second, reducing complexity is vital. By adopting new threading paradigms, developers can manage concurrency in a more intuitive way. For instance, using an actor model simplifies state management, which is often a source of bugs in traditional threading approaches. Working with actors allows for better modularity and maintainability in code.
Moreover, the ongoing trend towards cloud computing further emphasizes the need for efficient threading models. As applications increasingly rely on cloud resources, being able to perform operations concurrently across various nodes is essential.
End
The conclusion of this article serves as a critical point to reflect on the overall information presented regarding threads in computing. Understanding threads is essential for IT professionals and tech enthusiasts for various reasons. It encapsulates the software development paradigm and illustrates core principles that govern concurrent programming.
In particular, threads facilitate enhanced performance optimization by allowing multiple operations to occur simultaneously. This ability significantly improves the responsiveness and speed of applications. Recognizing this, developers leverage threads to create efficient systems that handle large volumes of concurrent tasks. Furthermore, discussing types of threads—user threads, kernel threads, and hybrid threads—equips readers with a nuanced perspective on how various threading models impact application architecture and performance.
Challenges associated with thread programming also merit attention. Debugging multithreaded applications presents unique difficulties that can mislead programmers who are less familiar with concurrent execution models. This understanding is paramount for mitigating performance bottlenecks and creating robust software solutions.
Additionally, future trends highlighted throughout this article signal the ongoing evolution in threading technology. Emerging paradigms and innovations are shaping software development. Thus, staying informed about these developments is important for IT professionals seeking to create competitive applications.
Ultimately, the essence of comprehending threads lies in their intricate relationship with modern computing. Their role is imperative not just in performance but also in influencing the future of technology.
Knowledge of threads becomes indispensable in crafting applications that function efficiently in a multithreaded environment.
Key Takeaways
- Threads Enhance Performance: Utilizing threads allows programs to execute tasks in parallel, improving overall execution speed.
- Types of Threads: A foundation in user, kernel, and hybrid threads helps developers employ the right threading model for their needs.
- Challenges in Thread Programming: Acknowledgment of issues like race conditions and deadlocks is essential for effective debugging and development.
- Future of Threading Technology: Continuous evolution in threading paradigms indicates the necessity for programmers to adapt and stay informed.
- Core Understanding Matters: Grasping the concept of threads is vital to navigating today's complex computing landscape.